๐ React Final Form HTML5 Validation
You can now get native HTML5 validation functionality by substituting your Field component with one from react-final-form-html5-validation.
Validation is probably the most complicated part of maintaining form state: deciding when to validate values, and when and where to display error messages are important design decisions for making your forms as useable as possible by the widest range of users.
Over a decade ago, the W3C set out some guidelines for what they thought the next version of HTML should look like, and they called it HTML5. Part of that standard included a system for validating form fields, both with native browser rules, and also the ability to set a custom validation error on a field. These days, the HTML5 validation standard is more-or-less supported by all web browsers.
Why use HTML5 Validation?
I see three primary reasons why HTML5 form validation is a good thing to incorporate into your application:
Consistency of Design
By using the browserโs built-in mechanism for displaying form errors, your users will be given a consistent UI for form errors across the entire internet (or at least those very few sites that are advanced as yours will be when you start using HTML5 form validation).
Accessibility
This is the big one. Screen readers and other accessibility tools need a lot of help understanding what exactly went wrong when a form submission fails. Some screen readers will also let the user know a field is required when they enter the field for the first time.
Built-In Focus On Errors
As of last week, ๐ Final Form supports a plugin to
focus on the first form field with an error. But with HTML5 Form Validation, it
comes for free from the browser! The browser stops the form submission and
focuses on the first error even before React is given the onSubmit
event.
Introducing...
๐ React Final Form HTML5 Validation
Iโve written a drop-in replacement for the Field
component in ๐ React Final
Form that wraps the official Field
component and adds the additional HTML5
validation onto it by doing three things:
- Deducing which DOM input corresponds to the field
- Injecting a special field-level validation function that reads from the
input.validity
DOM structure. - Setting the HTML5 validation error with
input.setCustomValidity()
if there is an error from a specified field-level validation function.
The result is a two-way binding between the browser native HTML5 Validation, and
any field-level validation function specified to the component. The bindings are
two-way because any
HTML5 contraint validation errors
will be added to the ๐ Final Form state, and any field-level validation
errors from ๐ Final Form will be set into the HTML5 validity.customError
state. Unfortunately, this functionality is not compatible with ๐ React Final
Form record-level validation, so the two should not be mixed.
โWhy not add this functionality directly into the officially bundled
Field
component?โ
Good question. The reason is that not everyone needs this functionality, and not
everyone is using ๐ React Final Form with the DOM (e.g. some people use it with
React Native). Therefore it makes sense to make this a separate package. This
version of Field
is a thin wrapper over the official Field
component, and
the only Field
API that this library uses/overrides is the field-level
validate prop, so even if you are using this libraryโs Field
component, you
will still get improvements as features are added to the ๐ React Final Form
library in the future.
"How to use it?"
Well, first you change this:
import { Field } from "react-final-form";
to this:
import { Field } from "react-final-form-html5-validation";
All the regular API of Field
stays the same, except that now you can pass in
props like maxLength={25}
or required
and, optionally, messages to display
when those rules are broken, e.g. tooLong="Be more concise!"
or
valueMissing="You need to answer this"
respectively. All of these prop names
are taken from the
HTML5 Specification,
so if you have staff already trained to the standards, they should pick it up
right away.
What does it look like?
Well, the error UI look slightly different in every browser. No doubt the users of each of these browsers will recognize this format of displaying error messages.
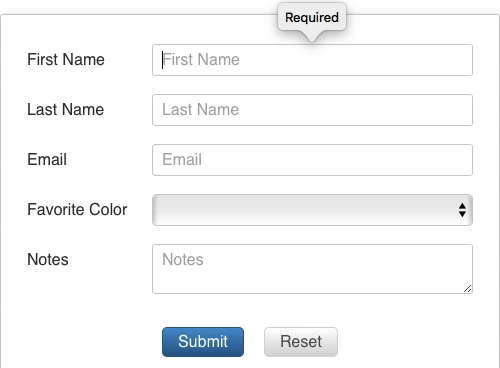
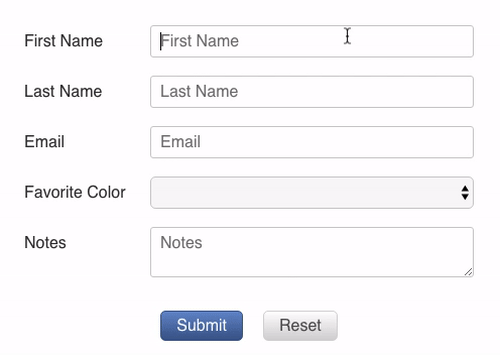
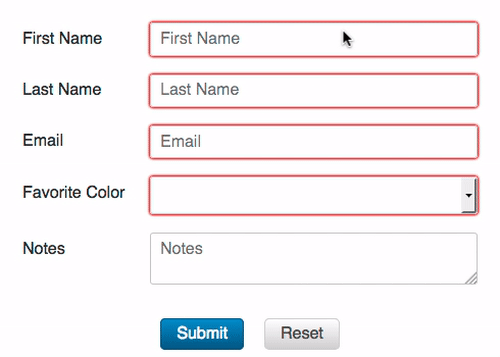
And hereโs the obligatory sandbox embed for you to play withโฆ
Conclusion
HTML5 Form Validation is yet another step towards making ๐ Final Form the most versatile, extensible form state management framework for React and beyond. Just think how pleased your boss will be when you inform her that youโve made all your forms much more accessible! ๐
โค๏ธ Thanks for reading. โค๏ธ